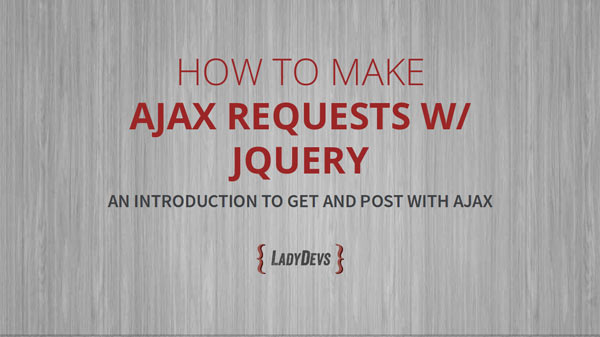
Want to walk through building an Ajax GET and POST request? I'll show you how to use jQuery to Ajaxify a webpage, and we'll use the data to add elements to the DOM. You'll get to know the $.ajax and $.get methods, and increase your familiarity with jQuery in general.
Watch on Vimeo Get the slidesTranscript
How To Make AJAX Requests with jQuery - An introduction to GET and POST with AJAX
Dated: 2017-07-13. Transcribed: 2017-07-13.
Intro
Hey everybody, welcome back. Today we're going to continue our web development fun train and we're going to learn to make AJAX requests with jQuery. We're going to be making both GET and POST requests today with AJAX.
I know you want to learn AJAX! It's so cool. It sauces it up. But in case you wanted me to spell it out for you, AJAX will give your websites a really cool, sleek, and seamless experience.
Here on the left (photo in slides) we have the old way that books were printed. Things were set letter by letter. You could still do it that way - some people still do for artisanal reasons - but that's the old way. That's in the past. Likewise, AJAX is the new way to do it. It's smooth and improved, and there are a lot of benefits.
On the right (photo in slides) we have a nice hotel room opening because you want to give all of your website users a sparkling, wonderful, and interesting experience that leaves them with a very positive impact from your website. You want them to find your website very intuitive, and AJAX will help you do that.
So anytime you want to avoid that page reload, look to AJAX. Anytime you want to grab some data and build the page after its already started to load - AJAX! If you want to make your UI more interactive, more like a native app or a piece of software - AJAX!
Anytime you've submitted a form and it's told you, Hey - your email is already in the database. Or, We don't recognize that email address. Well you were using ajax, because it was pinging the server and finding out if that email address was there, and returning that data. So you are already using ajax as a user whether you realized it or not.
You might be asking WHAT is ajax? It's kindof a mouthful, it stands for Asynchronous Javascript And XML. What you need to know is that it is a set of technologies that work together and this is what lets you grab other things invisibly behind the scenes for your users, while they are on the page. Maybe a JSON feed or another entire webpage. Whatever.
We're going to use jQuery today because it's going to handle all the cross browser issues but if you get a little deja vu, that's because we have already used ajax before with XMLHttpRequest. We used that for our Chuck Norris Joke Generator, and we really did grab that after the page loaded, so that was ajax.
The reason we're going to go with jquery today is because it is really easy to read and write. It better be, right? That's kindof their motto. Write less and do more. It's a very popular library. Any time you spend improving your jQuery skills is time well spent. There are a lot of projects that use it, it is very widespread. The community is great, the docs are great. And to include it in our project we'll just do one line of code. A single link to a CDN and we've got it.
So let's do it! You're going to need a text editor today, or if you want you can just follow along with me and just watch what I am doing on the screen.
We are going to make both a GET and a POST request today with ajax. And there are some starter files for this project. So get your self a directory where you can execute this on a server environment. I've got one (showing /var/www/jquery-ajax on screen). And you just jump on over to Github, and remember you do not have to have a github account to use this. Just click on the green button and you can download it.
I'm going to open it up, and you can drag over all the files if you like. But I am going to drag over just these four. These are the really essential files today. (skipped files: readme, scripts_complete.js)
Aaand let's go ahead and look at the files in this project. We have an index file. That is where all of our HTML is going to live. It is just 50... Not quite 50 lines long, and let's just run through it really quickly.
Here at the top we are grabbing a CRSF token, that is for the security of our POST request that we're going to be making. We're going to let PHP create a token there for us and store it in a cookie. We have the HEAD element with our title and our call to a Bootstrap theme to make things look good without having to try too hard. We have just a couple more styles.
If you're familiar with the Bootstrap style markup you'll see we have a header and the rows, containers, columns, and all that. The other element on the page is this select dropdown box with a couple of options.
The last thing that's on the page is actually hidden right now, that is a form with a hidden input for that CRSF token. And we have an empty div with an id of products. That is where jQuery is going to put all the products that we get back from our GET request.
The (truly) last thing on the page are the scripts. We put the scripts at the bottom so that they load last, and everything else is hopefully ready when they load. We've got jQuery from a CDN, and we've got our scripts.js file.
Let's go ahead and open scripts.js. You can see I've started you out with an almost-blank document here. We're going to put all of our jQuery today right inside these two wrapper elements. The outermost one is going to alias this long name "jQuery" into this short character: $. This is very handy. And this second one is going to say, Don't run a line of jQuery - don't do a single thing - until the document is totally ready in the browser.
We do have two more scripts in our foldere here today. We have a GET handler, that's where we're going to send our GET request. And we have a POST handler, this is where we'll send our POST request. The GET file I've named get-api.php because while it is on our site today, it doesn't have to be on our site. We could be grabbing this from somewhere else.
So that's what we've got. I hope you've got it!
The very first thing.... Actually, let's go ahead and look at that page in the browser. Here's what we've got, and our flimsy pretense today is that we are The Image Store, and we sell images. I guess they would be curated images, because here you can choose different sets of images, depending on which of these options you choose from the dropdown.
So I'm going to open up the console, and we just looked at the HTML together for this page. There is no magic going on, and you can see when I choose from the dropdown nothing happens in the console. So the very first thing we're going to do is change that. We're going to use jQuery to detect the dropdown being changed.
So here's the very first thing we're going to put into scripts.js. We're going to start our jQuery and put the element we want to target right inside these first parens. This is going to be a select element with the ID of "your-industry". When that element changes value -- when somebody chooses a dropdown option -- we are going to output to the console "You changed the dropdown to:" and then it's going to say what the value they chose was.
And let's just make sure that we have the indent type set to 2 spaces today, to keep everything consistent with the presentation slides.
jQuery and Ajax #1 Let's Detect the Dropdown Choice
So we're going to save that and we're going to go back to our Image Store in the browser. Just a fair warning, today we are going to hit some of the rocks and bumps on the way down together. We'll do a lot of things right but we're going to take our time to get there and talk about error handling.
Hit F5, and now when I click the dropdown you can see that jQuery is detecting that change/event, grabbing the value, and putting it into our console.log statement for us. If you got this, that's great!
#2 Set Up a $.get Call
The next thing we want to do is start setting up our GET request, and jQuery has this really great little function. It's just $.get, it's really short and easy. They call it a shorthand function because it is just so easy, and I really like it.
What we're going to do here is feed it only one thing: a URL. I've saved our URL in a variable. We're going to make the GET request and it's going to put anything that back into this variable called "data".
We have the first arguement here which will be the URL, and the second arg will be the callback. So what we're going to do is just console.log everything that comes back. We'll replace that prior console.log statement.
Save it and jump back on over to The Image Store. Hit F5. And now, when I choose an industry, Hm... okay, this is interesting right? I'm getting something (screen shows json with success: false). We're getting a string here that looks like JSON, doesn't it? We're getting a message that says success was false, and the msg is Unauthorized.
Okay, this is something.
Ajax Get Request #3 Start the Query String
What we need to do is start building that query string. If you remember, all of the GET request are passed on a long thing that is appended to the filename or URL. Remember, when you see the question mark - you know that's a GET request, and everything after are key and value pairs.
So I will tell you right off the bat, because I built the frontend and the backend of this, what it wants from us... It's telling us we are not authorized because it is looking for a key. That should actually be called "key" and the value needs to be "my-api". So let's go ahead and add some stuff on the back of that query string. We'll also pass the "industry", which we can get because we are inside the select element by using $(this).val(). It's going to be the value of dropdown option.
We'll go ahead and stick that on the end -- so now the URL is of course the get-api.php file, and we've also added some variables. This is how we're goin to pass our rudimentary security key, "key", to the script file.
Hit F5 and try the dropdown again. Remember, last time it said Success: False, Unauthorized.
Something different has happened! This is good. We now have Success: False, and the message is Invalid Request. Well something is happening, right?
I like things that don't fail silently. I don't know about you, but I like things that give me a unique message and a place to start debugging.
#4 Parse JSON if Needed, Show Error
So like I said, we're going to hit a couple bumps on the way down together, before it gets better. Let's start by taking that string and parsing it into real JSON in case you weren't getting that already. We're going to do that here by detecting if the data was a string, and if so, turn it back into JSON.
Then we will have access to all those properties and methods that might be on that data object.
Now we want to detect that Success property, and we'll say if it was false -- if this whole thing was not successful -- let's add an error message on the screen. We're going to go ahead and add that in. We'll remove that other console.log and make the indents pretty.
So we're going to try to detect success, and remember we are not successful yet, so what should happen is that data.msg... If a message was provided, it will be put into an error element. And if no data.msg was provided, we'll just get a generic error.
Let's go ahead and hit F5, try it again, and you can see we get Invalid Request on the screen. So we used to have a console.log statement here, for people like you and me who open their developer tools with F12. And now we have a statement on the screen.
You can decide for yourself if that's something you'd like to pursue in your applications. But I really like things that do not go down without a fight. Things that don't fail silently. I like to see a reason when things are failing, and you know what, your users do too.
I think the most maddening thing is when something fails and there is no message, no change, just fail. Just inactivity. That is very hard to handle.
#4.5 Add One More Param To Query String
We're going to fix that bump now, that Invalid Request. There is one more thing we need to add on the query string.
This API could potentially do a lot of things and it doesn't know which thing we want so. So we are going to tell it with this get_photos=true. I've split this onto two lines for readability today, and I'll just copy it over.
The important part of doing this red text is that now everybody knows what is going on, and I PROMISE YOU when people get in touch - maybe in the testing process or dog forbid when the site is live - when people tell you what is wrong with this site, they will give you something unique and INVALUABLE to point to. They will not give you these horrible short "it didn't work" messages.
Those are the worst. When people can't tell you why it broke, and they don't tell you how to reproduce the problem.
All right! So now we have our URL that is a little bit longer now. It's going to the current directory plus get-api.php. We're telling it in the query string that the task we want to do is get_photos. We're passing the Key and the Industry. Save that, and let's see what the Image Store gives us this time.
Hit F5 and see what happens. I'll choose Advertising from the dropdown (and I did have to turn on my XHR requests in Console) and you can see, we just made a GET request!
We sent it get_photos=true, industry=advertising, key=my-api, and we did get back a response. We got back a JSON response (again). And it says success is true! And it has given us a new property called images, which is an array of unique IDs.
Ajax GET Request #5 We Are Expecting data.images
So we WERE expecting data.images actually, and that's what we're going to use to build our page. We really want it, so let's make sure in our code that we really did receive it. I'm going to go ahead and console.log that data element so we will see it in the next reload. That will be after it's turned into proper JSON of course.
And here what we're going to do is say that if Success was false, then add an error message to the page and return this whole thing. Just stop the whole train.
But now if Sucess was true, that's great! And we just want to make sure that the images were passed, because that's what we really want. So here I am going to say if images was not passed, there will be an alert.
I find that alerts are very easy to implement and very noisy. I like to use them sometimes for development only problems.
Let's see what happens when we reload The Image Store. Choose an industry aaaand... Great! We made our GET request (see console) and this is what it came back with. It's an object with images. So since we did get the images property, we didn't hit the error.
#6 Handle Successful Image Grab
Fantastic! Now what we need to do is actually use the data that we just grabbed. So what we're going to do is put them in the Products div that I showed you in the Index file. It's empty right now, but as people choose different things from the dropdown we will need to blank it.
So we'll reset its contents to nothing every single time. And we're going to loop over these IDs of these images, and set up a little bit of HTML. We'll have a couple of these per row (col-md-4). We'll put an image. These image ID's are all from Unsplash.com. They have great lovely royalty free stock photos.
We'll show the image from Unsplash and also a form element with a checkbox. The value will be that unique image ID. And underneath we'll just Say - because this is the image store - "Buy this image for $1". These images are not for sale, they are royalty free, but this is our flimsy premise, remember.
So then once we create this little snippet of HTML we are going to use this $.append() method in jQuery, and that means to stick it inside the element at the very end. And once that is all done we're going to find the closest form element, which you may remember is actually hidden, and we'll fade that in.
Misc code formatting banter
Go ahead and save that. Notice that we are doing a little bit of detection here to make sure that the data we got back is what we need to handle building our page and such. We'll get the products element ready (blank it), we'll build the HTML, then we'll stick it in the products div and show it to the user.
So let us together check out The Image Store - F5 - wonderful! We just made our first GET request in AJAX with jQuery! It has returned us these IDs, and we've built something on the page. Now we have ourselves a little image store, don't we!
Now what we need to do is actually make something happen when we place the orders. (screencast shows form submit fail). So nothing is set up right now on this form. We're going to want to submit it with ajax.
Hopefully you got the same thing that I did, which is that you saw three images and every time you choose from the dropdown you get three new images. You get some checkboxes and this big Place Order button. None of that was on the page before, remember. That's all coming over and being added.
#7 Detect Form Submit Event
So now what we need to do is target this button right here (place order). We need to detect when the user clicks that, so that we can make our POST request.
The next thing to add, just like we did for the SELECT element, but for the FORM element.
Formatting banter... I like things lining up, yes I do
So here we are targeting the only form on the page. If this was a page with more going on, we would need to put an ID in there, but today this is the only one on the page.
You'll notice there is one extra thing with a Form .submit(), which is that it will pass the event into the callback function, and you must always (if you want to use Ajax) catch it, and write this statement. Whatever the variable is named, write it first and then put dot preventDefault(). That's going to prevent the form from actually submitting and reloading the page.
What we'll do now is catch that form submit event, and stop it from happening, and we'll echo out to the console "You tried to submit the form." Go ahead and try it in the browser.
You will need to select an industry and some images, and then hit Place Order. And you can see that jQuery is catching our form submit. That's really good.
Ajax POST Request #8 Prepare the Data to Send
The next thing we need to do is prepare the data to send. You will remember last time we just added it onto the URL in a query string, but we don't do that with POST requests. So here we're going to set up an object, I'm going to call it post_vars.
We're going to pass that CRSF token - that's for security, remember - and jQuery is going to find that hidden input on the page and assign the value of it to this property. We're going to start an empty array of items. And we're going to, kind of like before with getphotos=true, we told the script handling this form that out of all the things it might do, this is what I want you to do right now. So we'll say ajaxorder=true.
Then right after that we're going to use jQuery to find all of the inputs with the name images[] that were actually checked. You can see here (screenshot of page) that these all have the same name of images, all of these checkmarks. And so it is going to figure out which ones the user has checked, and it's going to drop them in our items array.
So I'm going to put this code in and remove the console.log. You are going to want to leave in this e.preventDefault(), that's got to stay there.
#9 Let's Create the $.ajax Request
We've set up all our post variables to make our POST Ajax request, now let's actually do it.
We're going to use a different method now, we already used $.get which was a shorthand method, and this time we are going to use the full banana $.ajax method. And it looks like this (screen of code).
You're going to pass it an object full of properties and methods, and then afterwards you can chain some things on. Here we have chained on .fail(), and this means that if the endpoint was unavailable, then something would happen (alert('Ouch!')). This will happen sometimes... Not really that often, but I like to detect it.
Like I said before, I like things to fail loudly. Hopefully a fail() is something your users will not experience. If they did it would probably be due to downtime.
Up here in the object that we're going to pass in first we are going to give the type of request, because you can do both GET and POST. If you were looking at a rest API there could be even more. We're going to give it the URL of the place to handle this, which is going to be post-handler.php.
We're going to pass in those variables that we just prepared. And then there is going to be a success function which means that the data came back - if you got that page and didn't hit a 500 error - then this is what's going to happen.
That doesn't mean that your whole operation was a success. But it does mean that you didn't have a broken endpoint.
We'll stick this request right after our nice prepared page_vars object, and you'll notice we are just going to console.log whatever comes back. Or if it fails, we'll get an alert.
So let us refresh, choose a dropdown, choose some images, and make our fake purchase. And you can see that we made our GET request, we made our POST request, and we got back a JSON object. Well we got back a string that we'll turn into a JSON object. And it says success is true, and is giving us a msg property with what looks like some HTML that says "thank you this is your receipt".
That's wonderful! We had a success. Let's take it to the next step.
#10 Did We Get What We Expected?
I always like to make sure that we actually got what we expected. Sometimes for reasons unknown, you will not. So let's continue with our error handling in case something had gone wrong in that request.
We are going to make the data in a JSON object again, if somehow it came over as a string, and we're going to look at that data.success property again.
Now you could output this to the screen or you can make an alert. It's really up to you how much you want to handle that and what you want to do there. We're doing it a couple of different ways today because... Why not?
But I do recommend that you put some error handling in there because things do not always go the way you expected. And in those debugging moments you will wish that something was happening on the screen that somebody could point to or give you a little message or something.
#11 Handle Successful Submit
The other thing we're going to want to handle now is a successful submit. So as you saw, we did have a successful submit. We're doing everything right. We've got all our ducks in a row, and we want to take this receipt like element, and show it on the screen.
So we are handling a bad request up here if success was false, and down below we want to show the receipt. If for some reason we didn't get a data.msg, we'll make some little message first. When you go and use new variables you want to make sure they exist first, otherwise you can get a nasty error.
So we'll hide the form, and then right after the form we are going to pop in this HTML code that we have received back from the server. We're also going to hide that selection choice because at this point the user has already placed their order.
I'm going to take out that last console.log because that is over now, and that's it! This is our GET and POST request thing.
Let's see it in action. Hit F5. See that there is nothing on the page. Hit the server after the page loaded with the GET request, see the images load from Unsplash. We're going to choose a couple and hit that place order button.
You can see it made our POST request, and the server is going to do whatever that server side code is going to do. Process a payment, send an email, what have you, and it has returned back already. So fast. Like lightening. It says, Thank You This is Your Receipt and it gave us this html receipt which we displayed.
That's our tiny app!
#12 Test in Your Browser
I hope you got the same results that I did today in your browser and developer tools.
Wrap Up
Next time we can look at what's going on behind the scenes in those PHP scripts when they get our requests.
So I KNOW there is a lot of cool stuff you could be using AJAX for. The answer is always Yes, and you might wonder if you have to know what all the stuff means. Specifically asynchronicity, and xml, and Javascript.
You don't! And I think that's what's great about jQuery, it makes it really easy and you don't have to understand very much Javascript at all really. You just have to learn the syntax and have a basic understanding of what's going on. And then learn by doing, fiddle around with it.
The next thing I want you to try is working some Ajax requests into your own projects. If you want to give this presentation you are more than welcome to.
I hope you enjoyed building this little app with me and I would love to hear about it. I would love your feedback if you would like to give me stars or upvotes, emails or shares, comments what have you. Carrier pigeon? Please do, I would love it.
Have a great Thursday everybody and I'll see you next week.